What Will You Learn?
In this comprehensive Python full-stack course, you’ll master Python programming, including syntax and advanced concepts. You’ll develop full-stack skills with HTML, CSS, JavaScript, and frameworks like Django and Flask. The course covers database integration, focusing on design, SQL, and ORM concepts. You’ll also learn RESTful API development and create scalable web applications. Additionally, you’ll build responsive front-end designs using modern frameworks and client-side scripting, while embracing agile development practices.
Course Flow
The Python Full Stack Developer course begins with Python fundamentals, progressing to full-stack
web development with HTML, CSS, and JavaScript. Participants explore database integration,
master Python web frameworks like Django or Flask, delve into RESTful API development, and
refine front-end skills. The course also covers version control, deployment strategies, and agile
development practices.
Course Content for L1
Module 1: Bootstrap
- Introduction to Bootstrap Bootstrap Setup Bootstrap Containers Bootstrap Grids Bootstrap Tables
- Bootstrap Buttons, Navbars, Alerts Bootstrap Carousel
- Bootstrap Forms
Module 2: Angular JS
- Introduction to Angular Environment Setup Installing Angular CLI
- Directory Structure of Angular Fundamentals Angular Building Blocks Angular Data Binding
- String Interpolation Directives and Pipes Forms
- Approaches (Driven & Reactive) Validators
Module 3: JavaScript
- Introduction to JavaScript How to Apply JavaScript
- Displaying Output in JavaScript Understanding JavaScript Syntax Variables & Datatypes Operators
- Math and String Manipulations Conditional and looping Statements Functions
- Validations
- Events
Module 4: Introduction to Python
- What is Python and history of Python Unique features of Python
- Python-2 and Python-3 differences Install Python and Environment Setup First Python Program
- Python Identifiers, Keywords and Indentation Comments and document interlude in Python Command-line arguments
- Getting User Input Python Data Types What are variables?
- Python Core objects and Functions Number and Maths
- Assignments
Module 5: Control statements
- if-else
- if-elseif-else while loop for loop break continue assert
- pass return
Module 6: List, Ranges & Tuples In Python
- Introduction Lists in Python More about Lists
- Understanding Iterators
- Generators, Comprehensions and Lambda Expressions
- Generators and Yield Next and Ranges
- Understanding and using Ranges More About Ranges
- Ordered Sets with tuples.
Module 7: Python dictionaries and sets
- Introduction to the section Python Dictionaries
- MORE ON DICTIONARIES SETS
- Python Sets Examples Input and Output in Python
- Reading and writing text files writing Text Files
- Appending to Files and Challenge Writing Binary Files Manually Using Pickle to Write Binary Files
Module 8: Python built in function.
- Python user defined functions Python packages functions
- Defining and calling Function The anonymous Functions
- Loops and statement in Python Modules & Packages
Module 9: Python object oriented.
- Overview of OOP The self-variable Constructor
- Types Of Variables Namespaces
- Creating Classes and Objects Inheritance
- Types of Methods Instance Methods Static Methods Class Methods Accessing attributes
- Built-In Class Attributes
- Destroying Objects
- Abstract classes and Interfaces Abstract Methods and Abstract class Interface in Python
- Abstract classes and Interfaces
Module 10: Exceptions
- Errors in Python Compile-Time Errors Runtime Errors Logical Errors
- What is Exception?
- Handling an exceptiontry….except…elsetry-finally clause.
- Argument of an Exception Python Standard Exceptions Raising an exceptions
- User-Defined Exceptions
Module 11: Python regular expressions
- What are regular expressions? The match Function
- The search Function Matching vs searching Search and Replace
- Extended Regular Expressions Wildcard
Module 12: Python Multithreaded programming
- What is multithreading?
- Difference between a Process and Thread Concurrent Programming and GIL
- Uses of Thread Starting a New Thread The Threading Module
- Thread Synchronization Locks
- Semaphore Deadlock of Threads Avoiding Deadlocks Daemon Threads
- Using Databases in Python Python MySQL Database Access
- Install the MySQLdb and other Packages Create Database Connection
- CREATE, INSERT, READ Operation
- DML and DDL Oepration with Databases Web Scraping in Python
Module 13: Data science using python
- NumPy
- Introduction to NumPy Creating arrays Indexing Arrays
- Array Transposition Universal Array Function Array Processing
- Array Input and Output Matplotlib: Data Visualization Python for Data Visualization
- Welcome to the Data Visualization Section Matplotlib
- Pandas
Module 14: Graphical user interface
- Python GUI Frameworks
- Django overview Creating a project Apps life cycle Admin interface Creating views URL Mapping Template system Models
- Sessions, caching and comments RSS,AJAX
- File uploading Cookie handling
Module 15: Framework for web development DJANGO
- Django overview
- Creating a project Apps life cycle Admin interface Creating views URL Mapping Template system Models
- Form details
- Page redirection Sending Emails
- Deploying Django framework Form processing
Module 16: Database
- Database handling with mysql python mysql database access create database connection
- Dml and ddl operations with databases performing transactions
- Handling database errors disconnecting database
- Database handling with mongodb sql vs nosql
- Mongodb pymongo
Course Content for L2
Module 1: Bootstrap
- Introduction to Bootstrap Bootstrap Setup Bootstrap Containers Bootstrap Grids Bootstrap Tables
- Bootstrap Buttons, Navbars, Alerts Bootstrap Carousel
- Bootstrap Forms
Module 2: Angular JS
- Introduction to Angular Environment Setup Installing Angular CLI
- Directory Structure of Angular Fundamentals Angular Building Blocks Angular Data Binding
- String Interpolation Directives and Pipes Forms
- Approaches (Driven & Reactive) Validators
Module 3: JavaScript
- Introduction to JavaScript How to Apply JavaScript
- Displaying Output in JavaScript Understanding JavaScript Syntax Variables & Datatypes Operators
- Math and String Manipulations Conditional and looping Statements Functions
- Validations
- Events
Module 4: Introduction to Python
- What is Python and history of Python Unique features of Python
- Python-2 and Python-3 differences Install Python and Environment Setup First Python Program
- Python Identifiers, Keywords and Indentation Comments and document interlude in Python Command-line arguments
- Getting User Input Python Data Types What are variables?
- Python Core objects and Functions Number and Maths
- Assignments
Module 5: Control statements
- if-else
- if-elseif-else while loop for loop break continue assert
- pass return
Module 6: List, Ranges & Tuples In Python
- Introduction Lists in Python More about Lists
- Understanding Iterators
- Generators, Comprehensions and Lambda Expressions
- Generators and Yield Next and Ranges
- Understanding and using Ranges More About Ranges
- Ordered Sets with tuples.
Module 7: Python dictionaries and sets
- Introduction to the section Python Dictionaries
- MORE ON DICTIONARIES SETS
- Python Sets Examples Input and Output in Python
- Reading and writing text files writing Text Files
- Appending to Files and Challenge Writing Binary Files Manually Using Pickle to Write Binary Files
Module 8: Python built in function.
- Python user defined functions Python packages functions
- Defining and calling Function The anonymous Functions
- Loops and statement in Python Modules & Packages
Module 9: Python object oriented.
- Overview of OOP The self-variable Constructor
- Types Of Variables Namespaces
- Creating Classes and Objects Inheritance
- Types of Methods Instance Methods Static Methods Class Methods Accessing attributes
- Built-In Class Attributes
- Destroying Objects
- Abstract classes and Interfaces Abstract Methods and Abstract class Interface in Python
- Abstract classes and Interfaces
Module 10: Exceptions
- Errors in Python Compile-Time Errors Runtime Errors Logical Errors
- What is Exception?
- Handling an exceptiontry….except…elsetry-finally clause.
- Argument of an Exception Python Standard Exceptions Raising an exceptions
- User-Defined Exceptions
Module 11: Python regular expressions
- What are regular expressions? The match Function
- The search Function Matching vs searching Search and Replace
- Extended Regular Expressions Wildcard
Module 12: Python Multithreaded programming
- What is multithreading?
- Difference between a Process and Thread Concurrent Programming and GIL
- Uses of Thread Starting a New Thread The Threading Module
- Thread Synchronization Locks
- Semaphore Deadlock of Threads Avoiding Deadlocks Daemon Threads
- Using Databases in Python Python MySQL Database Access
- Install the MySQLdb and other Packages Create Database Connection
- CREATE, INSERT, READ Operation
- DML and DDL Oepration with Databases Web Scraping in Python
Module 13: Data science using python
- NumPy
- Introduction to NumPy Creating arrays Indexing Arrays
- Array Transposition Universal Array Function Array Processing
- Array Input and Output Matplotlib: Data Visualization Python for Data Visualization
- Welcome to the Data Visualization Section Matplotlib
- Pandas
Module 14: Graphical user interface
- Python GUI Frameworks
- Django overview Creating a project Apps life cycle Admin interface Creating views URL Mapping Template system Models
- Sessions, caching and comments RSS,AJAX
- File uploading Cookie handling
Module 15: Framework for web development DJANGO
- Django overview
- Creating a project Apps life cycle Admin interface Creating views URL Mapping Template system Models
- Form details
- Page redirection Sending Emails
- Deploying Django framework Form processing
Module 16: Database
- Database handling with mysql python mysql database access create database connection
- Dml and ddl operations with databases performing transactions
- Handling database errors disconnecting database
- Database handling with mongodb sql vs nosql
- Mongodb pymongo
What Students Say...
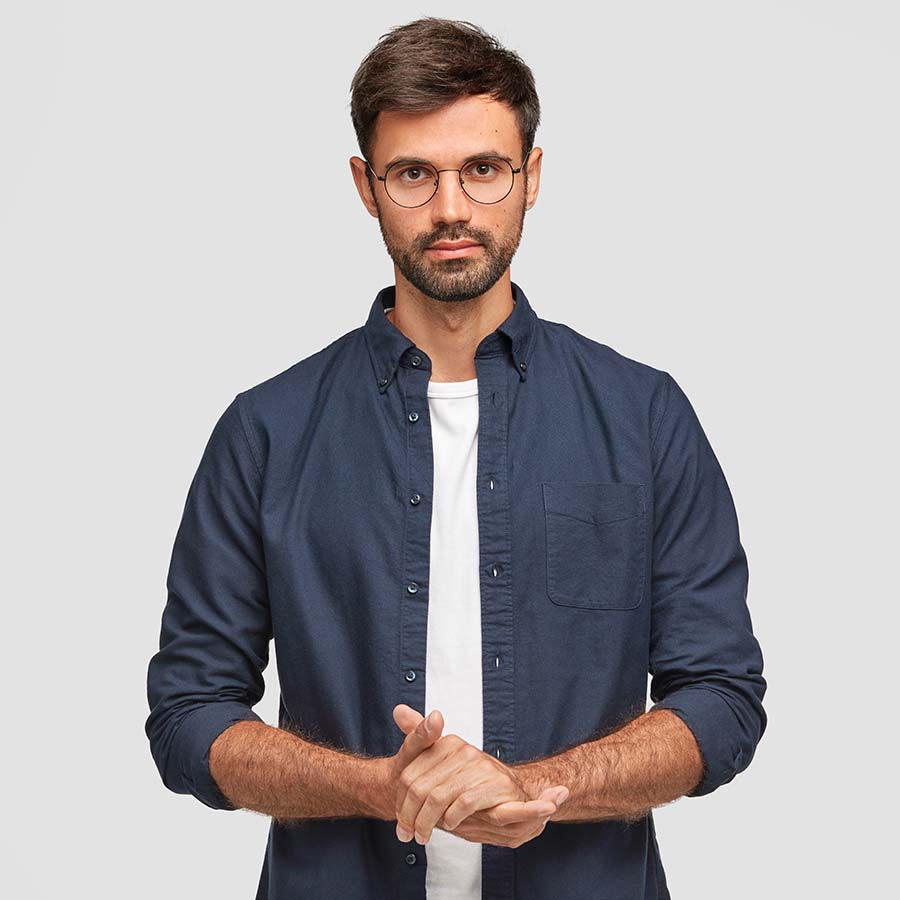
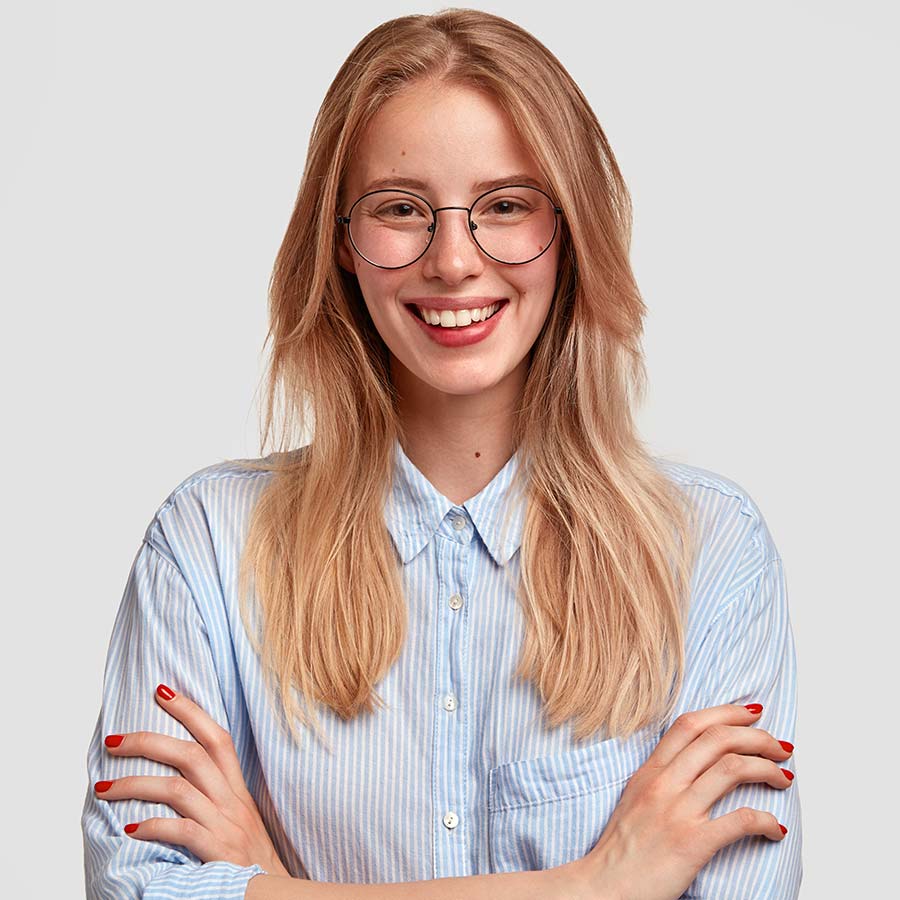
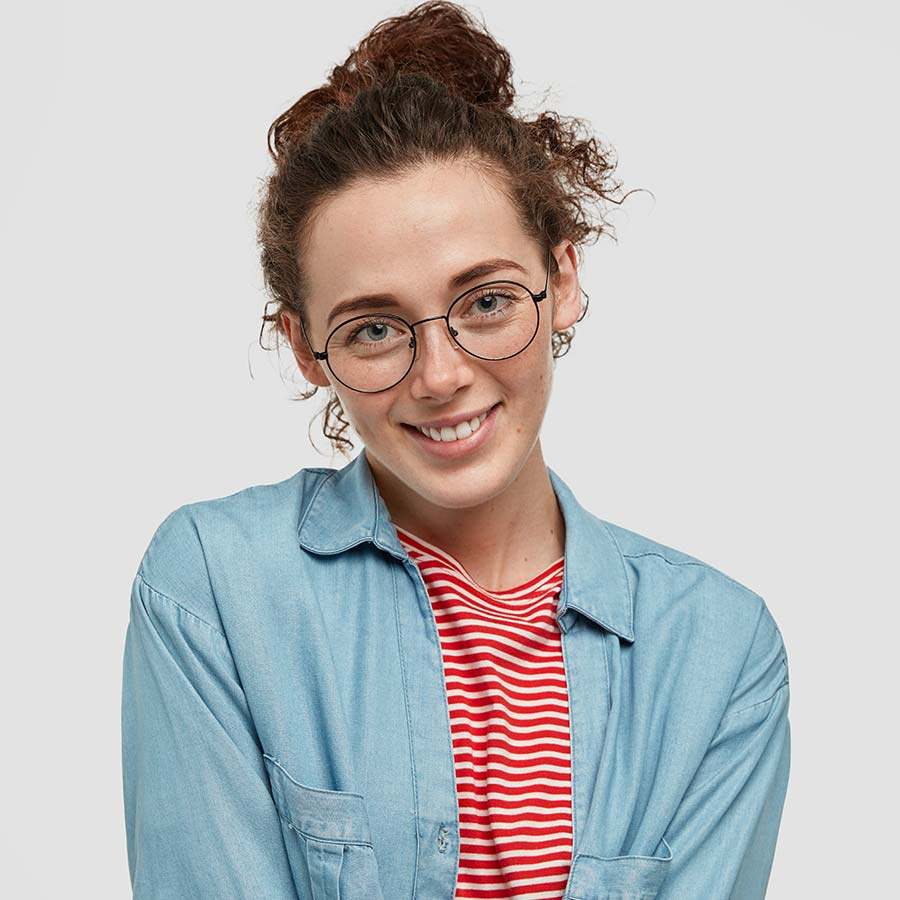
Certifications
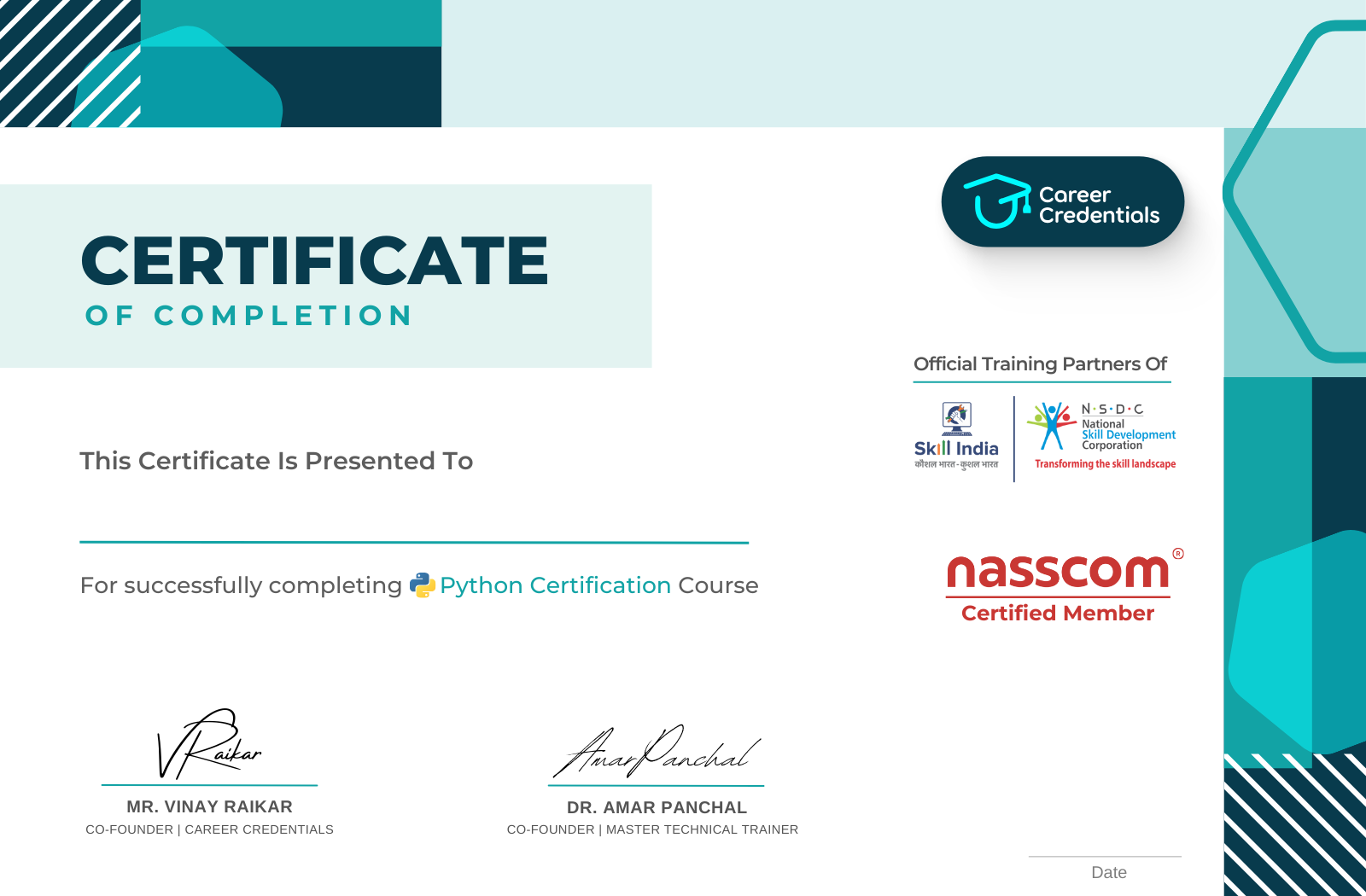
Career Credentials
Python Certified
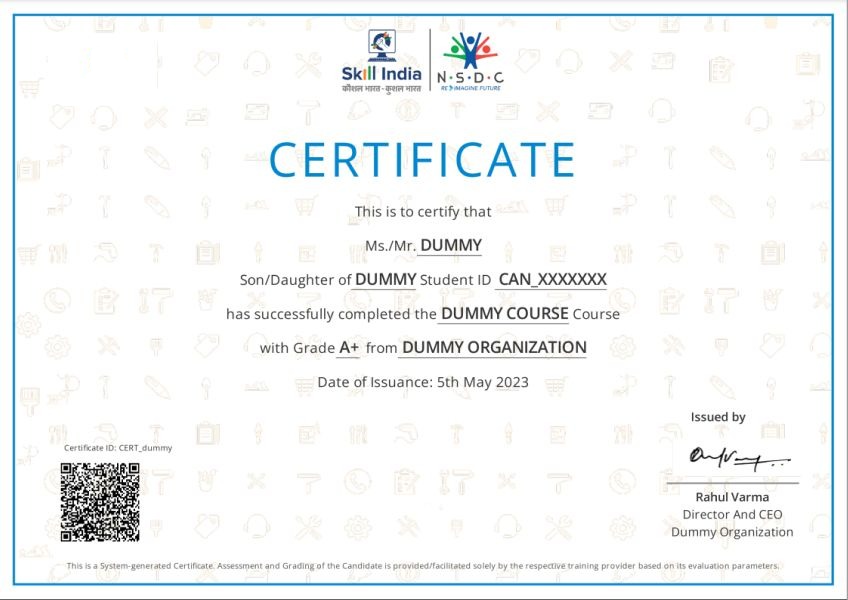
NSDC
National Certification
- Level
Beginner
- Total Enrolled
189
- Duration
6 Months
- Mode
Online
A course by
Material Includes
- Comprehensive Python programming tutorials covering syntax, data structures, and advanced concepts.
- Full-stack development resources with HTML, CSS, JavaScript, Django, and Flask.
- Database integration guides, including database design, SQL, and ORM concepts.
- RESTful API development and responsive front-end design materials, emphasizing agile practices.
Prerequisites
- Basic understanding of programming concepts.
- Familiarity with HTML, CSS, and JavaScript is recommended.
- A computer with internet access for coding and online resources.
- Willingness to learn and apply agile development practices.
Projects
- Chat GPT App System Design
- Personality Predictor Application
- Banking Application
Get Expert Career Guidance for Free!!!
Join us on Free 1:1 Counselling session where we provide personalised Career Guidance for Every Student